Enhancing API Test Clarity and Reporting with Junit and Allure: Leveraging Descriptive Names
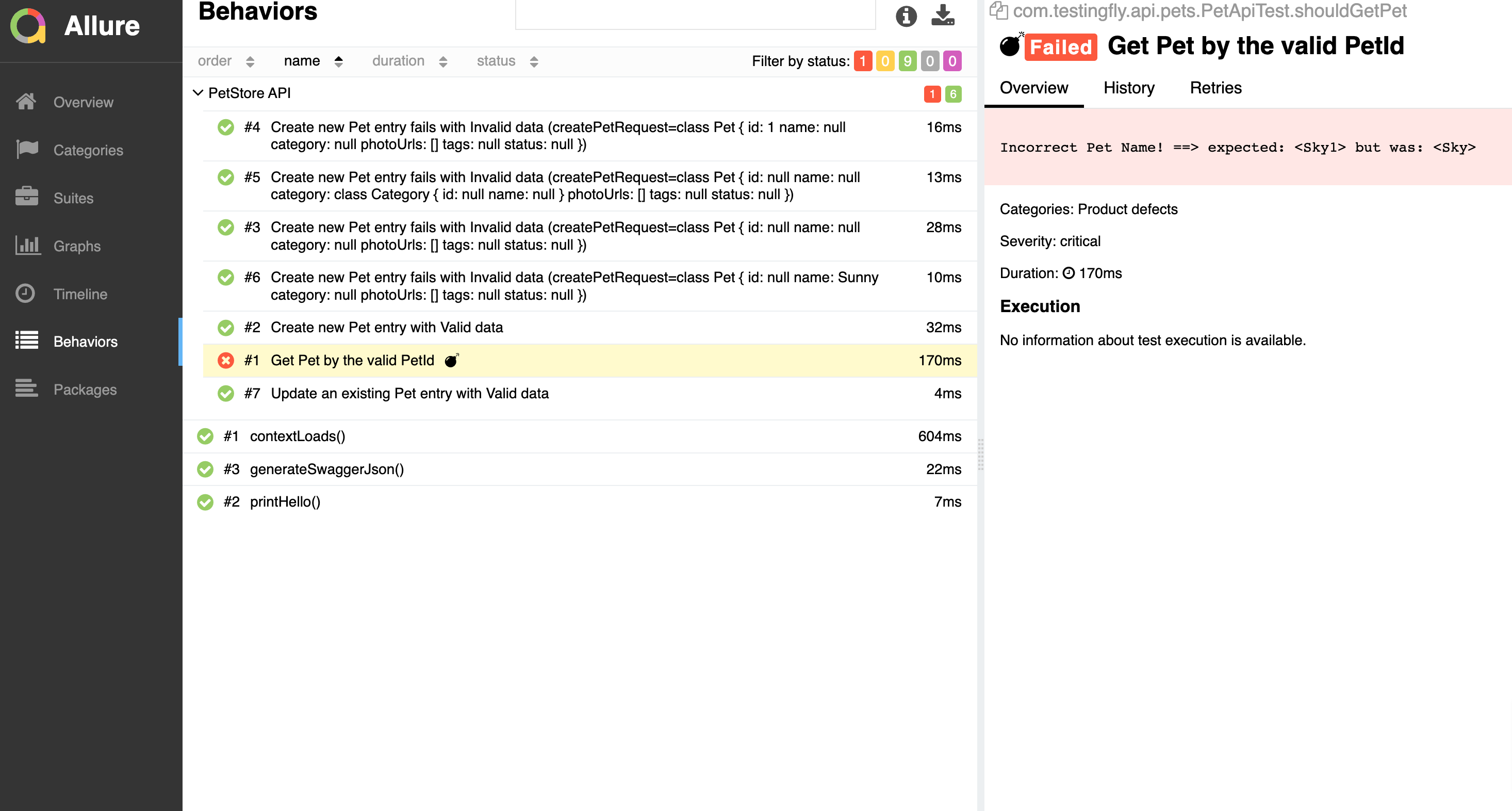
In this tutorial, we'll explore best practices for writing API functional tests using JUnit and enhancing test reporting with Allure. These best practices ensure that your tests are well-structured, meaningful, and easy to maintain.
Table of Contents
- Writing Meaningful Test Names for Enhanced Allure Reports
- Implementing the 3As Pattern
- Putting It All Together
- Conclusion
1. Writing Meaningful Test Names for Enhanced Allure Reports
Meaningful test names are essential for understanding the purpose of each test case, especially when considering the benefits they provide in Allure reports to end users. While it's a good practice to keep test method names short for developers, leveraging the @DisplayName
annotation to provide descriptive names enhances the value of test reports for end users.
Benefits of Descriptive Names in Allure Reports:
-
Improved Readability: End users can quickly grasp the purpose and context of each test case without digging into the test code.
-
Clear Documentation: Descriptive names serve as self-documentation, reducing the need for additional documentation outside the codebase.
-
Faster Debugging: In case of test failures, descriptive names pinpoint the exact scenario being tested, making debugging more efficient.
-
Better Collaboration: Team members, including non-technical stakeholders, can better understand and contribute to testing efforts with descriptive test names.
Examples:
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
public class APITest {
@Test
@DisplayName("Retrieve User Profile - Positive Scenario")
public void testGetUserProfilePositive() {
// Arrange
// Act
// Assert
}
@Test
@DisplayName("Create New User with Valid Data")
public void testCreateNewUserWithValidData() {
// Arrange
// Act
// Assert
}
// ... more test cases
}
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
public class APITest {
@Test
@DisplayName("Retrieve User Profile - Positive Scenario")
public void testGetUserProfilePositive() {
// Arrange
// Act
// Assert
}
@Test
@DisplayName("Create New User with Valid Data")
public void testCreateNewUserWithValidData() {
// Arrange
// Act
// Assert
}
// ... more test cases
}
Here's an example of the test result with the descriptive name:
> Task :integrationTest
User API > Create New User with Valid Data PASSED
BUILD SUCCESSFUL in 2s
3 actionable tasks: 2 executed, 1 up-to-date
> Task :integrationTest
User API > Create New User with Valid Data PASSED
BUILD SUCCESSFUL in 2s
3 actionable tasks: 2 executed, 1 up-to-date
2. Implementing the 3As Pattern
The Arrange-Act-Assert (3As) pattern provides a clear structure for writing tests:
- Arrange: Set up the necessary preconditions and test data.
- Act: Perform the actual API request or action you want to test.
- Assert: Verify the response or outcome to ensure it meets the expected behavior.
Structure your test methods using the 3As pattern:
@Test
@DisplayName("Retrieve User Profile - Positive Scenario")
public void testGetUserProfilePositive() {
// Arrange
// Act
// Assert
}
@Test
@DisplayName("Retrieve User Profile - Positive Scenario")
public void testGetUserProfilePositive() {
// Arrange
// Act
// Assert
}
3. Putting It All Together
By following these best practices, you create well-structured and maintainable API functional tests. Descriptive test names, along with the 3As pattern, improve the quality and understandability of your tests, benefiting both developers and end users.
4. Conclusion
In this tutorial, you've learned best practices for writing API functional tests using JUnit and enhancing your reports with Allure. These practices ensure your tests are not only reliable but also easily understandable for end users. Descriptive test names significantly contribute to improved communication and documentation in your testing efforts. Happy testing!
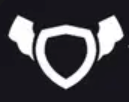
About Testingfly
Testingfly is my spot for sharing insights and experiences, with a primary focus on tools and technologies related to test automation and governance.
Comments
Want to give your thoughts or chat about more ideas? Feel free to leave a comment here.
Instead of authenticating the giscus application, you can also comment directly on GitHub.
Related Articles
Testing iFrames using Playwright
Automated testing has become an integral part of web application development. However, testing in Safari, Apple's web browser, presents unique challenges due to the browser's strict Same-Origin Policy (SOP), especially when dealing with iframes. In this article, we'll explore known issues related to Safari's SOP, discuss workarounds, and demonstrate how Playwright, a popular automation testing framework, supports automated testing in this context.
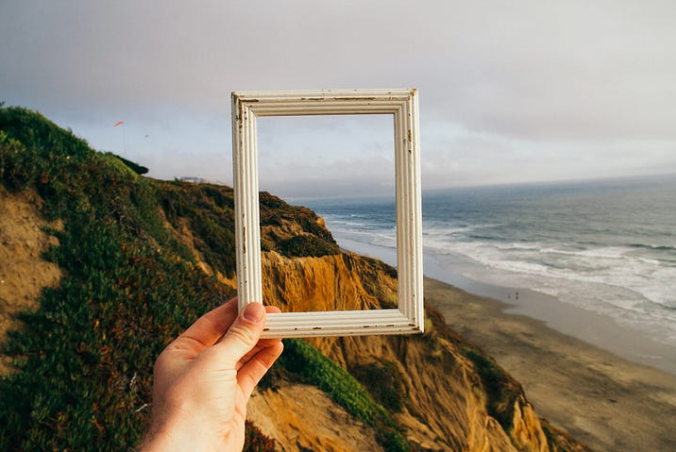
Overview of SiteCore for Beginners
Sitecore is a digital experience platform that combines content management, marketing automation, and eCommerce. It's an enterprise-level content management system (CMS) built on ASP.NET. Sitecore allows businesses to create, manage, and publish content across all channels using simple tools.
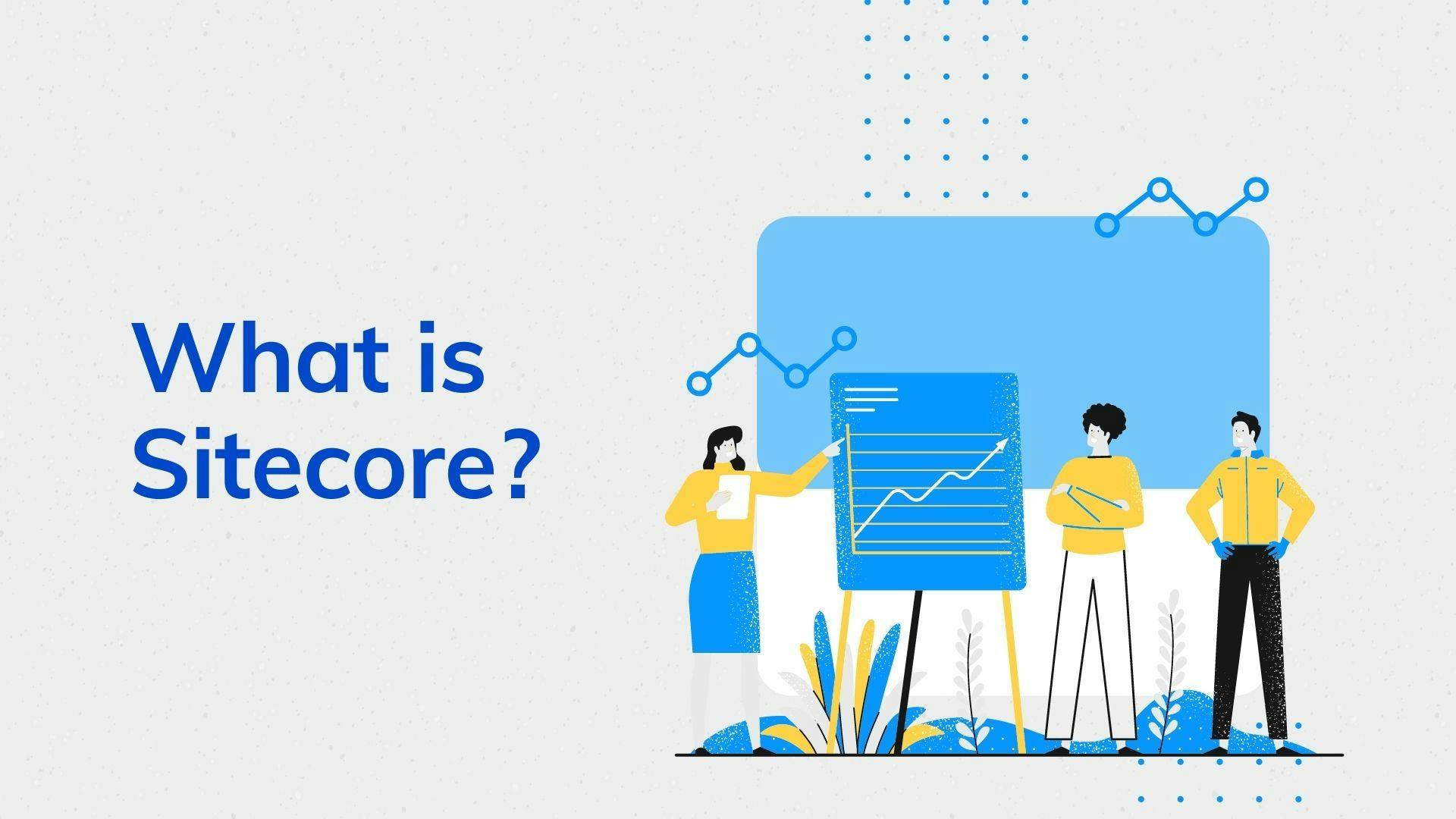