Enable efficient and maintainable UI test automation by incorporating 'data-testid' attributes
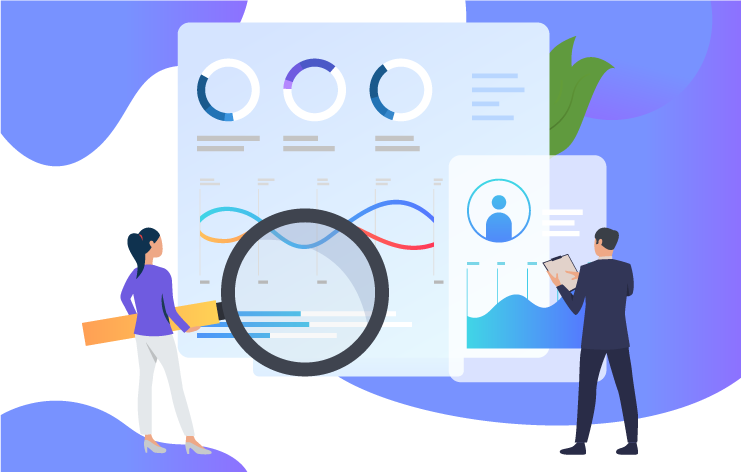
Objective:
- Enable efficient and maintainable UI test automation by incorporating “data-testid” attributes.
- Centralize the management of these attributes in a shared file for clarity, consistency, and ease of maintenance.
Steps:
1. Create a Centralized Locator File:
Create a dedicated file, e.g., locators.ts, to store all the “data-testid” attributes used for UI testing.
// locators.ts
export const LoginPage = {
usernameInput: '[data-testid="login-username"]',
passwordInput: '[data-testid="login-password"]',
loginButton: '[data-testid="login-button"]',
};
export const HomePage = {
welcomeMessage: '[data-testid="welcome-message"]',
userProfileLink: '[data-testid="user-profile-link"]',
};
// locators.ts
export const LoginPage = {
usernameInput: '[data-testid="login-username"]',
passwordInput: '[data-testid="login-password"]',
loginButton: '[data-testid="login-button"]',
};
export const HomePage = {
welcomeMessage: '[data-testid="welcome-message"]',
userProfileLink: '[data-testid="user-profile-link"]',
};
2. Integrate Locators in the UI (e.g. Angular) Components:
In each Angular component, import the relevant locators from the centralized file and use them to identify the elements.
// login.component.ts
import { LoginPage } from 'path-to-locators/locators';
@Component({
selector: 'app-login',
template: `
<input [ngModel]="username" (ngModelChange)="username = $event" [attr.data-testid]="LoginPage.usernameInput" />
<input type="password" [ngModel]="password" (ngModelChange)="password = $event" [attr.data-testid]="LoginPage.passwordInput" />
<button (click)="login()" [attr.data-testid]="LoginPage.loginButton">Login</button>
`,
})
export class LoginComponent {
username: string = '';
password: string = '';
login() {
// Implement login logic
}
}
// login.component.ts
import { LoginPage } from 'path-to-locators/locators';
@Component({
selector: 'app-login',
template: `
<input [ngModel]="username" (ngModelChange)="username = $event" [attr.data-testid]="LoginPage.usernameInput" />
<input type="password" [ngModel]="password" (ngModelChange)="password = $event" [attr.data-testid]="LoginPage.passwordInput" />
<button (click)="login()" [attr.data-testid]="LoginPage.loginButton">Login</button>
`,
})
export class LoginComponent {
username: string = '';
password: string = '';
login() {
// Implement login logic
}
}
Benefits:
-
Consistency and Readability:
- Uniformly structured locators enhance code readability and maintain consistency across the application.
-
Centralized Management:
- All locators are stored in one central file, promoting easy maintenance and updates.
-
Collaboration:
- Developers and QA engineers can collaborate effectively by referencing the same set of locators for both testing and development.
-
Avoidance of Confusing Names:
- Group locators logically in the central file to prevent naming conflicts and confusion.
-
Scalability:
- Easily scale to accommodate a large number of pages and elements with an organized and logical grouping of locators.
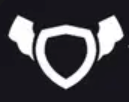
About Testingfly
Testingfly is my spot for sharing insights and experiences, with a primary focus on tools and technologies related to test automation and governance.
Comments
Want to give your thoughts or chat about more ideas? Feel free to leave a comment here.
Instead of authenticating the giscus application, you can also comment directly on GitHub.
Related Articles
Testing iFrames using Playwright
Automated testing has become an integral part of web application development. However, testing in Safari, Apple's web browser, presents unique challenges due to the browser's strict Same-Origin Policy (SOP), especially when dealing with iframes. In this article, we'll explore known issues related to Safari's SOP, discuss workarounds, and demonstrate how Playwright, a popular automation testing framework, supports automated testing in this context.
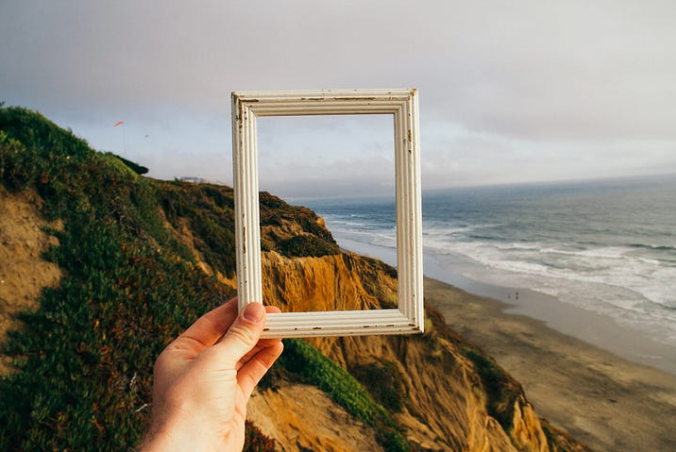
Overview of SiteCore for Beginners
Sitecore is a digital experience platform that combines content management, marketing automation, and eCommerce. It's an enterprise-level content management system (CMS) built on ASP.NET. Sitecore allows businesses to create, manage, and publish content across all channels using simple tools.
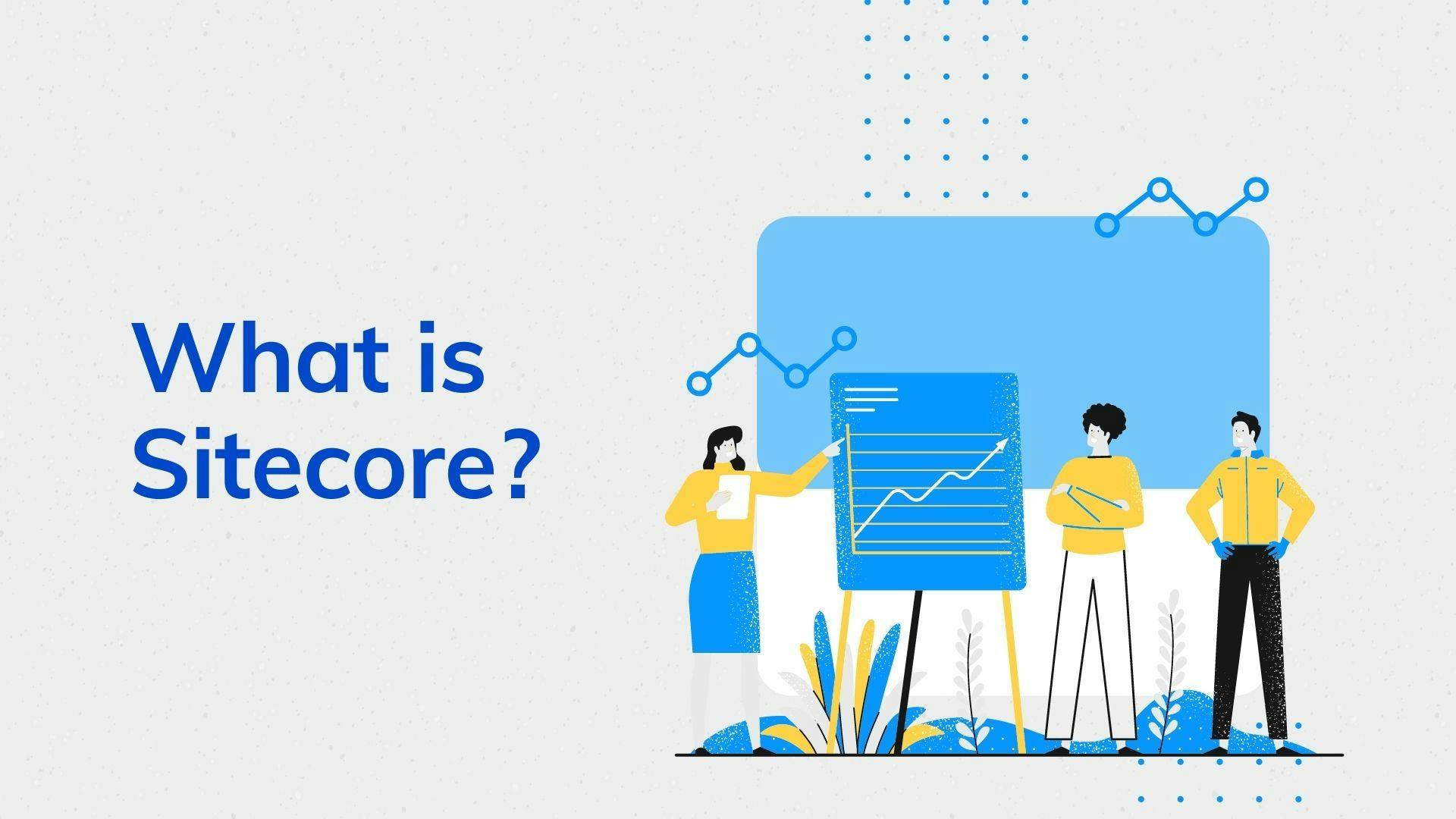