Relative Import vs. Absolute Import with Pytest: Best Practices
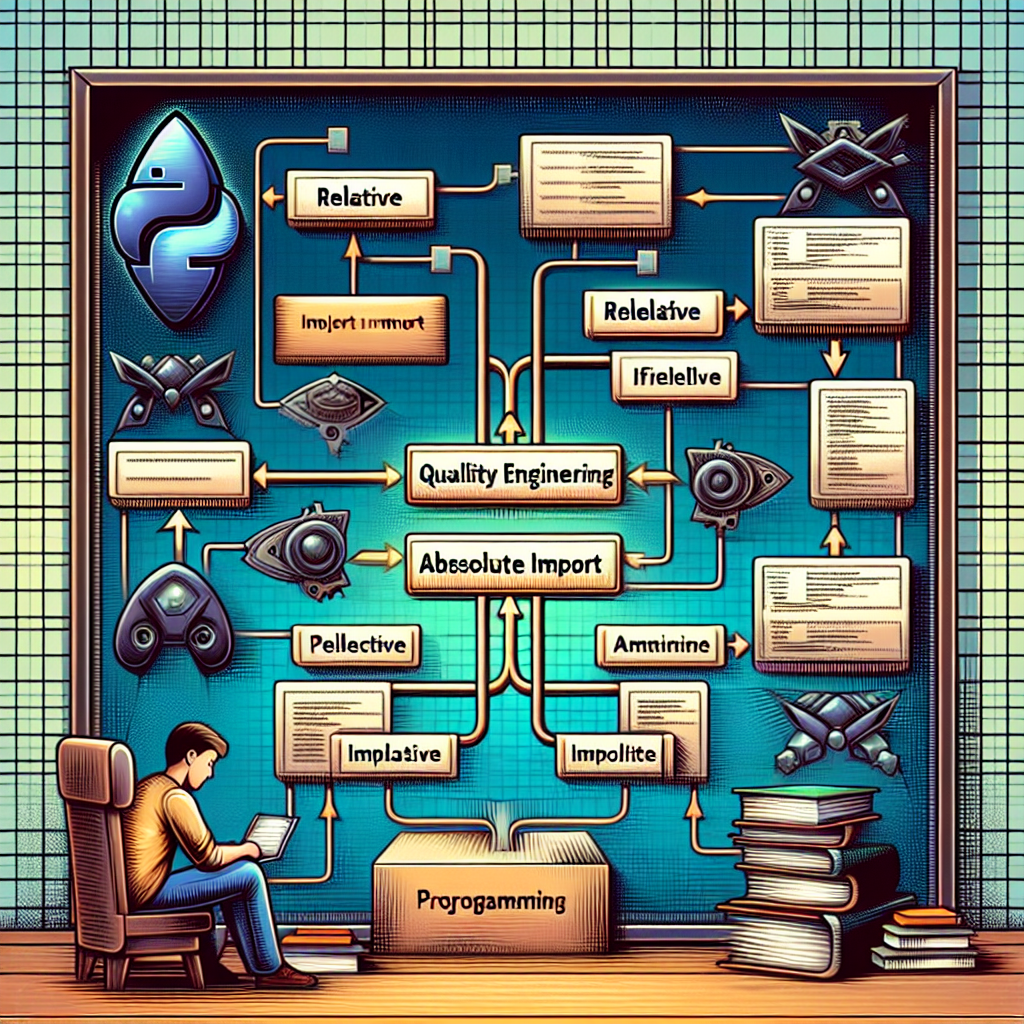
Table of Contents
- Introduction
- Relative Import vs. Absolute Import
- Execution as Script vs. Module
- When to Use Script vs. Module
- Common Pitfalls
- Conclusion
1. Introduction
When working with Python projects and writing tests with pytest, you'll often encounter choices regarding import styles and execution methods. Two key considerations are whether to use relative imports or absolute imports and whether to execute tests as a script or a module. Each approach has its advantages and pitfalls, and understanding when to use them is crucial for maintaining clean, reliable, and maintainable codebases. In this article, we'll explore these topics and provide guidance on best practices.
2. Relative Import vs. Absolute Import
2.1 Relative Import
Relative imports allow you to import modules or subpackages relative to the current module's location within a package. They use dot notation (.
) to specify the relative path to the desired module or package.
# Relative import example
from ..subpackage import module
# Relative import example
from ..subpackage import module
Best Practices:
- Use relative imports within packages to maintain code readability and avoid hardcoding package names.
- Ensure that the directory containing the module you want to import is a package with an
__init__.py
file.
2.2 Absolute Import
Absolute imports specify the exact location of the module or package to import using the full path from the project's root directory.
# Absolute import example
from your_package.subpackage import module
# Absolute import example
from your_package.subpackage import module
Best Practices:
- Prefer absolute imports for clarity, especially in larger projects or when importing modules from external packages.
- Avoid ambiguity by explicitly specifying the full path to the module or package.
3. Execution as Script vs. Module
3.1 Execution as Script
Executing pytest tests as a script involves running the pytest command directly in the terminal.
pytest test_module.py
pytest test_module.py
Best Practices:
- Useful for quick test runs during development.
- Works well for executing individual test files or subsets of tests.
3.2 Execution as Module
Executing pytest tests as a module involves using the -m
flag with the python
command.
python -m pytest test_module.py
python -m pytest test_module.py
Best Practices:
- Preferred method for running tests in automated CI/CD pipelines.
- Ensures consistent behavior across different environments.
- Facilitates better integration with test discovery mechanisms.
4. When to Use Script vs. Module
- Script Execution:
- Use when you need to run tests interactively or for ad-hoc testing.
- Suitable for development and debugging purposes.
- Module Execution:
- Use in CI/CD pipelines for automated testing.
- Preferred for consistent and reproducible test runs in different environments.
5. Common Pitfalls
- Using Script Execution for CI/CD: Running tests as a script in CI/CD pipelines can lead to inconsistent results due to differences in environment configurations.
- Misusing Relative Imports: Incorrectly structured packages or missing
__init__.py
files can cause relative imports to fail. - Relying Heavily on
sys.path
Manipulation: Modifyingsys.path
to enable imports can lead to maintenance issues and obscure dependency management.
6. Conclusion
Choosing between relative imports and absolute imports, as well as deciding whether to execute pytest tests as a script or a module, depends on factors such as project size, development workflow, and deployment environment. By following best practices and understanding the advantages and pitfalls of each approach, you can ensure that your codebase remains clean, maintainable, and conducive to effective testing.
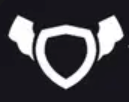
About Testingfly
Testingfly is my spot for sharing insights and experiences, with a primary focus on tools and technologies related to test automation and governance.
Comments
Want to give your thoughts or chat about more ideas? Feel free to leave a comment here.
Instead of authenticating the giscus application, you can also comment directly on GitHub.
Related Articles
Testing iFrames using Playwright
Automated testing has become an integral part of web application development. However, testing in Safari, Apple's web browser, presents unique challenges due to the browser's strict Same-Origin Policy (SOP), especially when dealing with iframes. In this article, we'll explore known issues related to Safari's SOP, discuss workarounds, and demonstrate how Playwright, a popular automation testing framework, supports automated testing in this context.
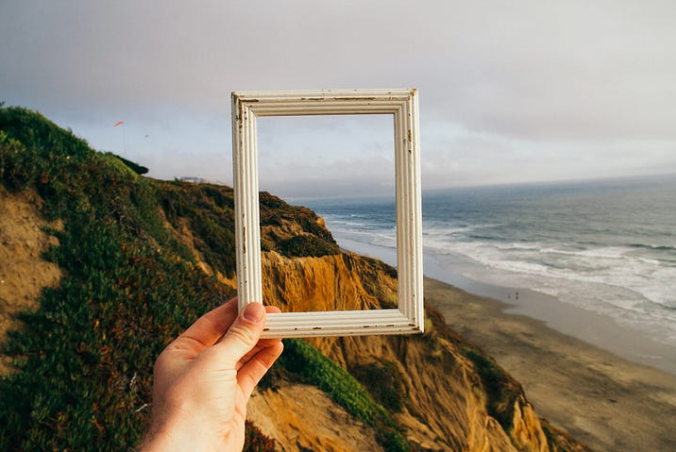
Overview of SiteCore for Beginners
Sitecore is a digital experience platform that combines content management, marketing automation, and eCommerce. It's an enterprise-level content management system (CMS) built on ASP.NET. Sitecore allows businesses to create, manage, and publish content across all channels using simple tools.
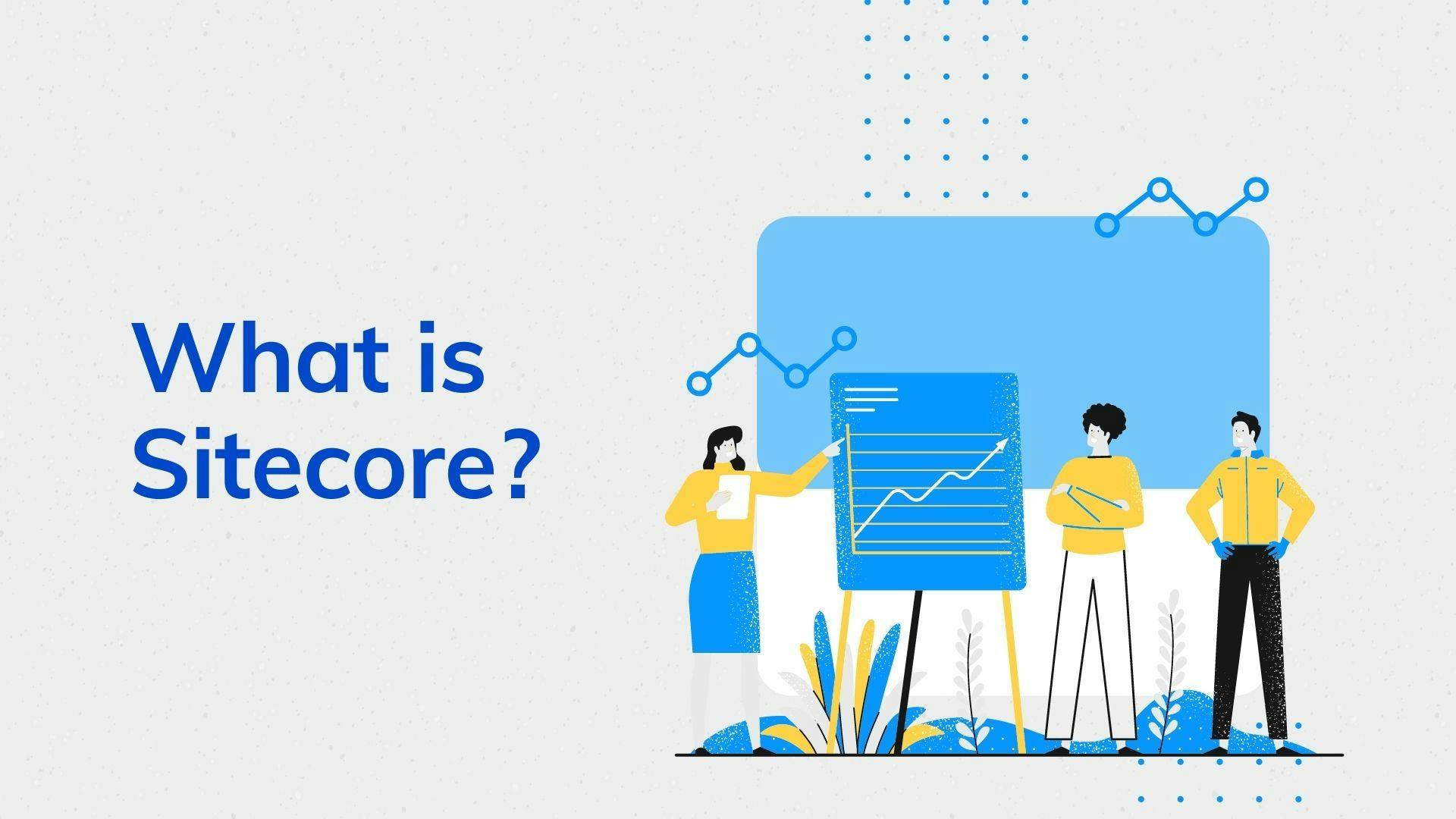